Finding entities
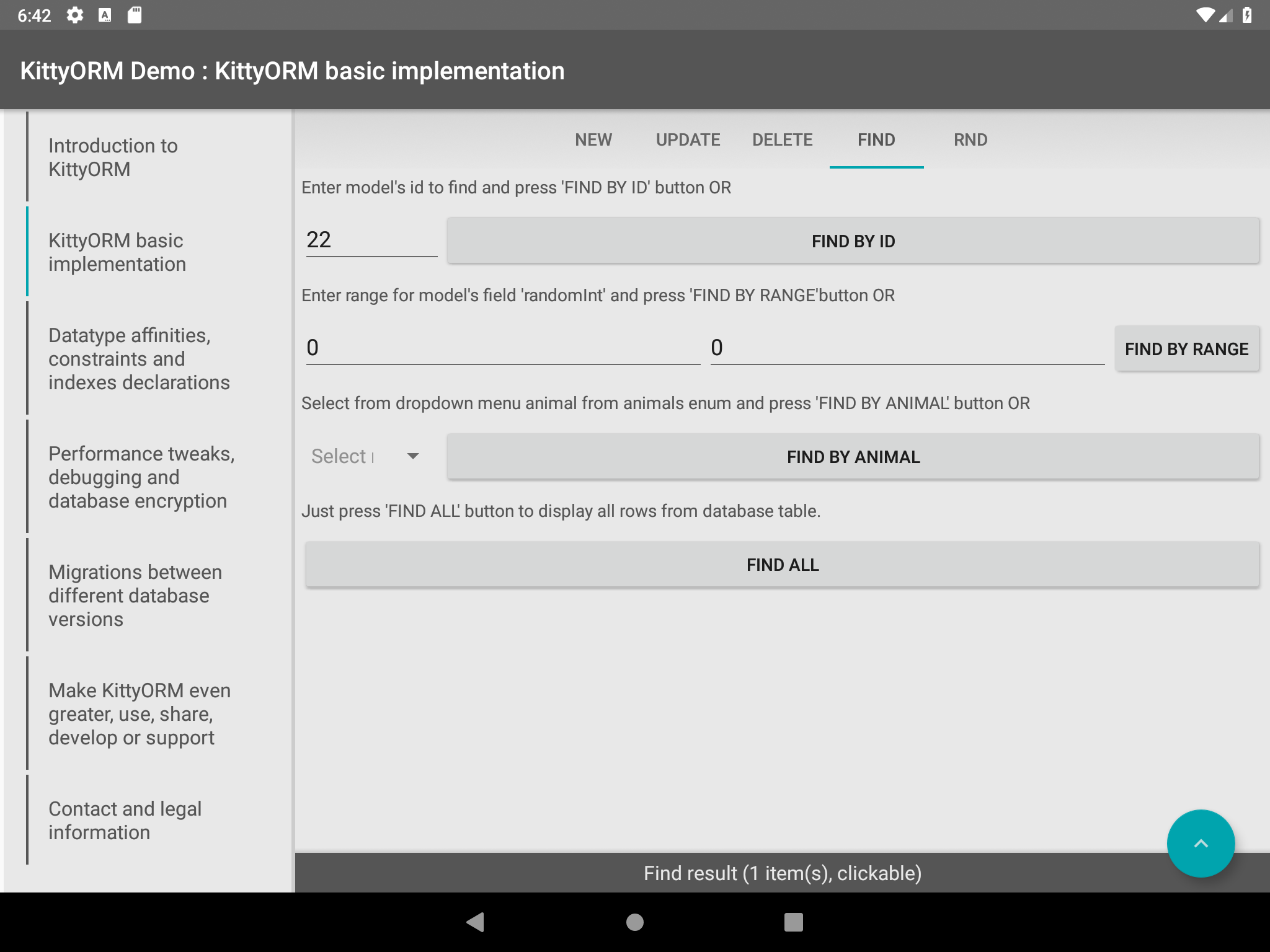
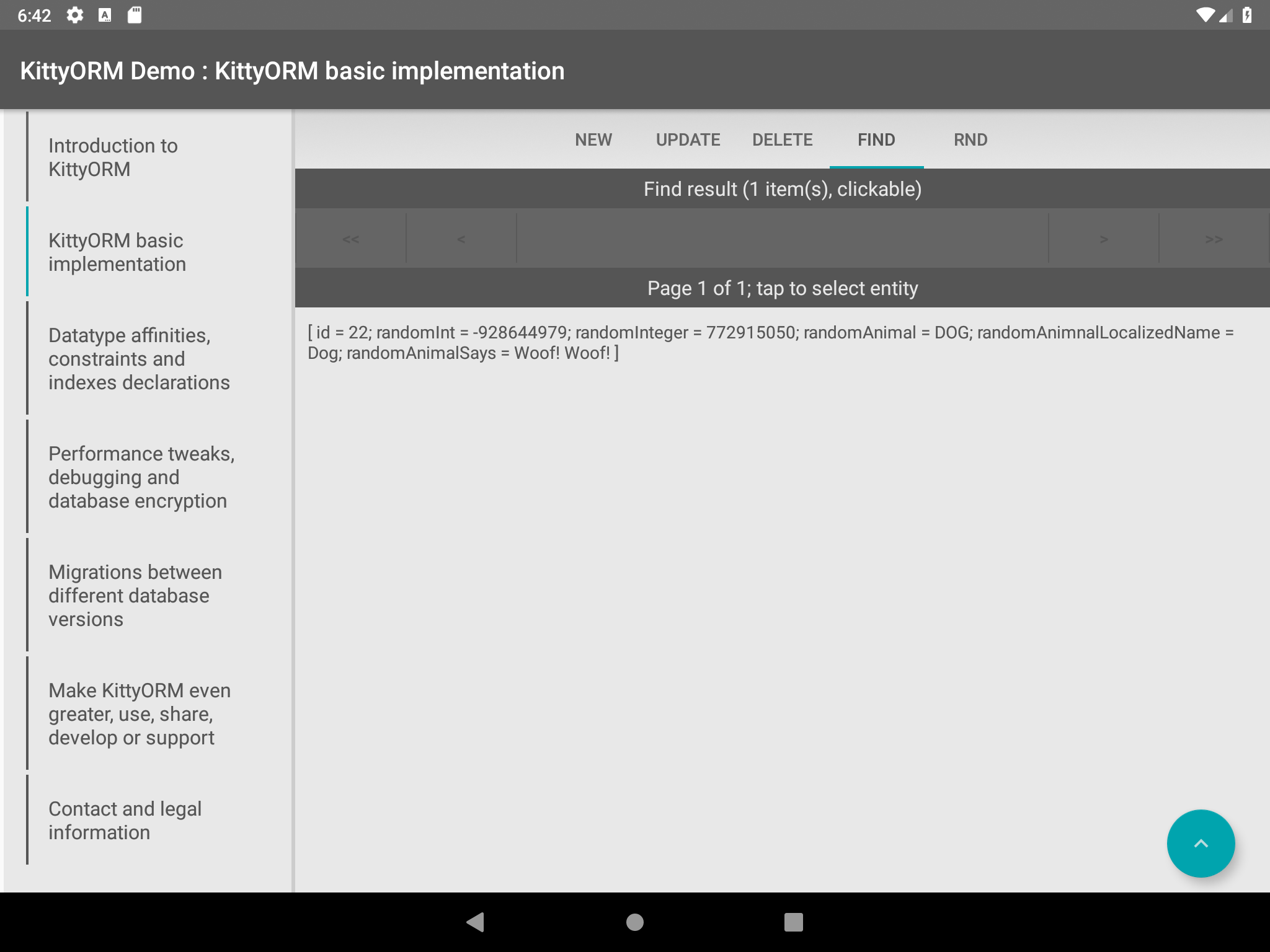
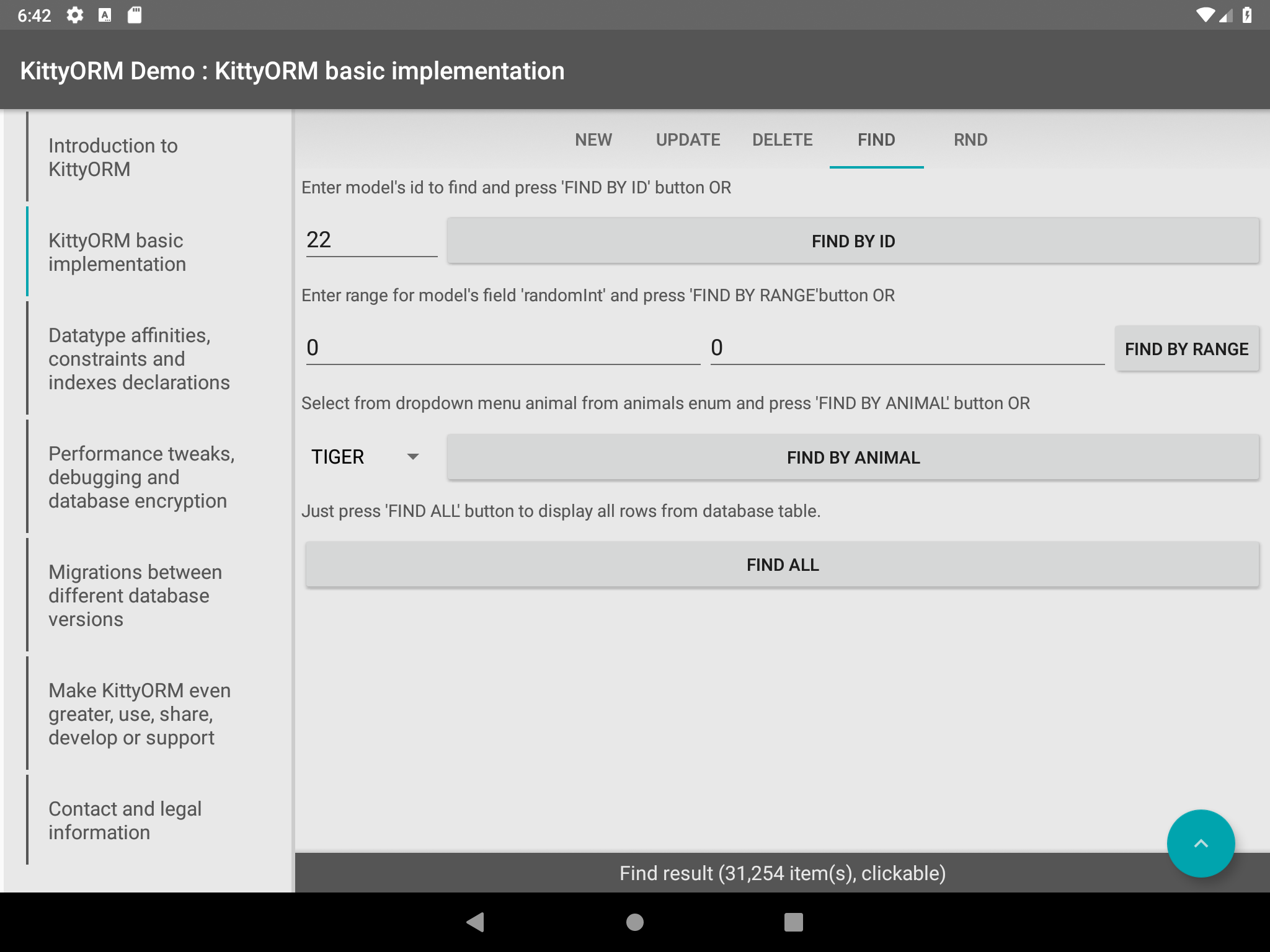
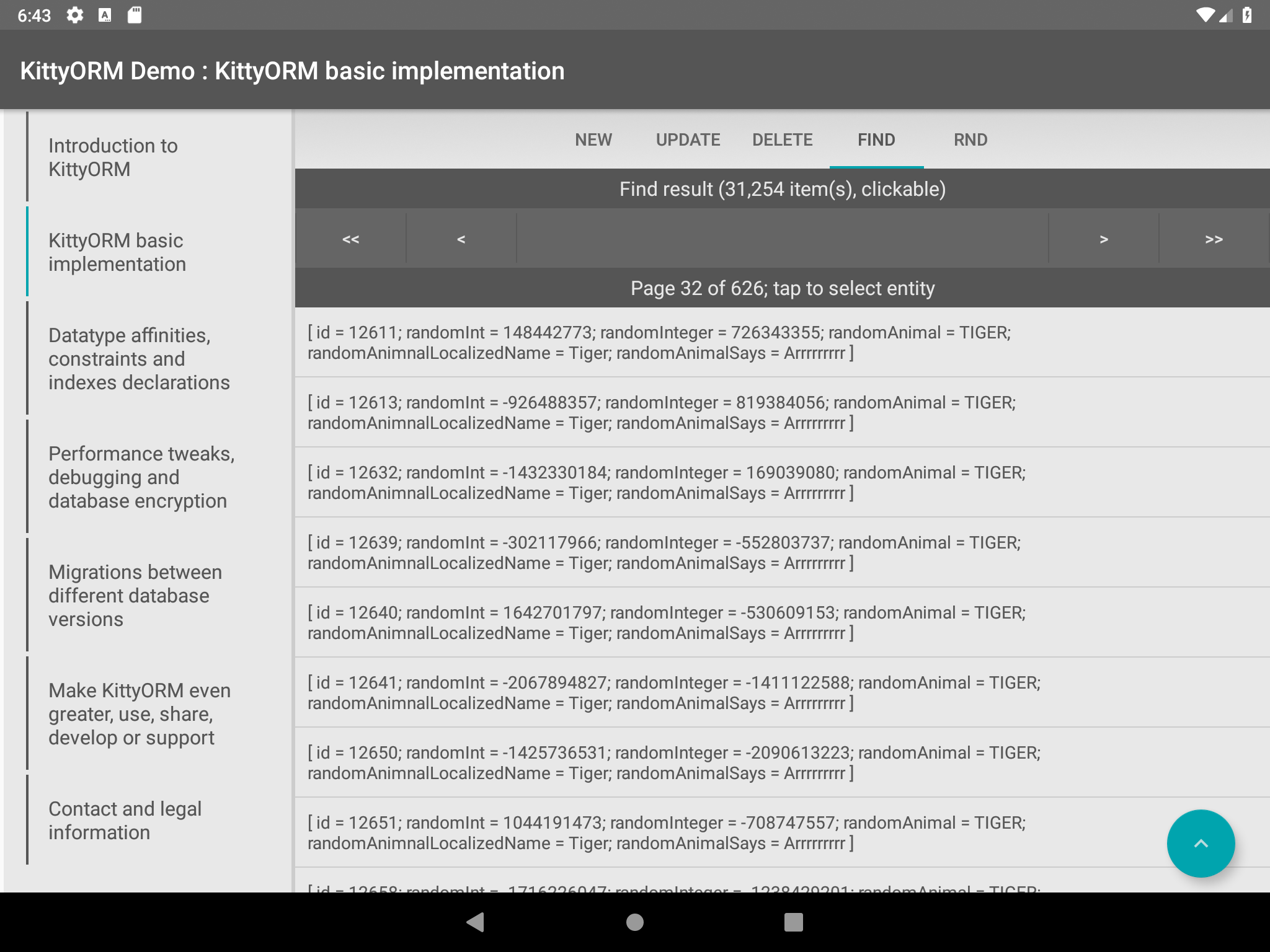
To find entities with KittyORM just use one of methods already implemented at KittyMapper.class
:
1// Initializing database instance
2BasicDatabase db = new BasicDatabase(getContext());
3// Getting mapper instance
4RandomMapper mapper = (RandomMapper) db.getMapper(RandomModel.class);
5// Getting existing model from database (assuming that 0l model exists)
6RandomModel byIPK = mapper.findByIPK(0l);
7// Getting existing model with rowid (assuming that 10l model exists)
8RandomModel byRowid = mapper.findByRowID(10l);
9// Getting all models
10List<RandomModel> all = mapper.findAll();
11// Getting model with condition (fetching 100 existing tigers)
12QueryParameters parameters = new QueryParameters();
13parameters.setOffset(0l).setLimit(100l);
14SQLiteConditionBuilder builder = new SQLiteConditionBuilder();
15builder.addField(AbstractRandomModel.RND_ANIMAL_CNAME)
16 .addSQLOperator(SQLiteOperator.EQUAL)
17 .addValue(Animals.TIGER.name());
18List<RandomModel> hundredOfTigers = mapper.findWhere(builder.build(), parameters);
19// Getting model with condition (fetching 100 existing tigers with SQLite string condition)
20hundredOfTigers = mapper.findWhere(parameters, "#?randomAnimal = ?", Animals.TIGER.name());
Already implemented find methods in KittyORM:
Method name | Method description |
---|---|
findWhere(SQLiteCondition where, QueryParameters qParams) |
Returns list of models associated with records in backed database table that suits provided clause and query parameters. |
findWhere(QueryParameters qParams, String where, Object... conditionValues) |
Returns list of models associated with records in backed database table that suits provided clause and query parameters. |
findWhere(SQLiteCondition where) |
Returns list of models associated with records in backed database table that suits provided clause. |
findWhere(String where, Object... conditionValues) |
Returns list of models associated with records in backed database table that suits provided clause. |
findAll(QueryParameters qParams) |
Returns list of all models associated with records in backed database table with usage of passed qParams. |
findAll() |
Returns list of all models associated with records in backed database table. |
findByRowID(Long rowid) |
Returns model filled with data from database or null if no record with provided rowid found. |
findByPK(KittyPrimaryKey primaryKey) |
Returns model filled with data from database or null if no record with provided PK found. |
findByIPK(Long ipk) |
Returns model filled with data from database or null if no record with provided IPK found. |
findFirst(SQLiteCondition where) |
Returns first record in KittyModel wrapper in database table that suits provided condition. |
findFirst() |
Returns first record in KittyModel wrapper in database table. |
findLast(SQLiteCondition where) |
Returns last record in KittyModel wrapper in database table that suits provided condition. |
findLast() |
Returns last record in KittyModel wrapper in database table. |
Do not count collection received from findAll()
method if you want to count all records in table, use already implemented sum and count methods!
See following count and sum code example snippet:
1// Initializing database instance
2BasicDatabase db = new BasicDatabase(getContext());
3// Getting mapper instance
4RandomMapper mapper = (RandomMapper) db.getMapper(RandomModel.class);
5// Count all records in database
6long count = mapper.countAll();
7// Count all dogs
8SQLiteConditionBuilder builder = new SQLiteConditionBuilder();
9builder.addField(AbstractRandomModel.RND_ANIMAL_CNAME)
10 .addSQLOperator(SQLiteOperator.EQUAL)
11 .addValue(Animals.DOG.name());
12long dogsCount = mapper.countWhere(builder.build());
13// Sum all dog's random_int
14long dogsRndIntSum = mapper.sum("random_int", "#?randomAnimal = ?", Animals.DOG.name());
Implementing extended CRUD controller
OK, this is simple, already implemented by KittyMapper
methods allows you to do most of the database related operations, however you may want to extend KittyMapper
CRUD controller and implement some methods that would be used commonly in your application. Here some steps to take:
Define in KittyORM registry CRUD controller to be used with particular model by defining it in your
KittyDatabase
implementation class with usage@KITTY_DATABASE_REGISTRY
atdomainPairs
or by defining it at your model implementation class with usage of@KITTY_EXTENDED_CRUD
annotation:1// Defining at registry example 2@KITTY_DATABASE( 3 databaseName = "basic_database", 4 domainPackageNames = {"net.akaish.kittyormdemo.sqlite.basicdb"}, 5 ... 6) 7@KITTY_DATABASE_REGISTRY( 8 domainPairs = { 9 @KITTY_REGISTRY_PAIR(model = ComplexRandomModel.class, mapper = ComplexRandomMapper.class), 10 @KITTY_REGISTRY_PAIR(model = IndexesAndConstraintsModel.class), 11 @KITTY_REGISTRY_PAIR(model = RandomModel.class, mapper = RandomMapper.class) // registry CRUD controller definition 12 } 13) 14public class BasicDatabase extends KittyDatabase { 15 ... 16} 17// Defining at model example 18@KITTY_TABLE 19@KITTY_EXTENDED_CRUD(extendedCrudController = RandomMapper.class) // model CRUD controller definition 20@INDEX( 21 indexName = "random_animal_index", 22 indexColumns = {AbstractRandomModel.RND_ANIMAL_CNAME} 23) 24public class RandomModel extends AbstractRandomModel { 25 ... 26}
Create new class that extends
KittyMapper.class
, implement default constructor and locate it at domain package, fill it with your logic.Click to view extended crud controller implementation example:
1public class RandomMapper extends KittyMapper { 2 3 public <M extends KittyModel> RandomMapper(KittyTableConfiguration tableConfiguration, 4 M blankModelInstance, 5 String databasePassword) { 6 super(tableConfiguration, blankModelInstance, databasePassword); 7 } 8 9 protected SQLiteCondition getAnimalCondition(Animals animal) { 10 return new SQLiteConditionBuilder() 11 .addColumn(RND_ANIMAL_CNAME) 12 .addSQLOperator("=") 13 .addObjectValue(animal) 14 .build(); 15 } 16 17 public long deleteByRandomIntegerRange(int start, int end) { 18 return deleteWhere("#?randomInt >= ? AND #?randomInt <= ?", start, end); 19 } 20 21 public long deleteByAnimal(Animals animal) { 22 return deleteWhere(getAnimalCondition(animal)); 23 } 24 25 public List<RandomModel> findByAnimal(Animals animal, long offset, long limit, boolean groupingOn) { 26 SQLiteCondition condition = getAnimalCondition(animal); 27 QueryParameters qparam = new QueryParameters(); 28 qparam.setLimit(limit).setOffset(offset); 29 if(groupingOn) 30 qparam.setGroupByColumns(RND_ANIMAL_CNAME); 31 else 32 qparam.setGroupByColumns(KittyConstants.ROWID); 33 return findWhere(condition, qparam); 34 } 35 36 public List<RandomModel> findByIdRange(long fromId, long toId, boolean inclusive, Long offset, Long limit) { 37 SQLiteCondition condition = new SQLiteConditionBuilder() 38 .addColumn("id") 39 .addSQLOperator(inclusive ? GREATER_OR_EQUAL : GREATER_THAN) 40 .addValue(fromId) 41 .addSQLOperator(AND) 42 .addColumn("id") 43 .addSQLOperator(inclusive ? LESS_OR_EQUAL : LESS_THAN) 44 .addValue(toId) 45 .build(); 46 QueryParameters qparam = new QueryParameters(); 47 qparam.setLimit(limit).setOffset(offset).setGroupByColumns(KittyConstants.ROWID); 48 return findWhere(condition, qparam); 49 } 50 51 public List<RandomModel> findAllRandomModels(Long offset, Long limit) { 52 QueryParameters qparam = new QueryParameters(); 53 qparam.setLimit(limit).setOffset(offset).setGroupByColumns(KittyConstants.ROWID); 54 return findAll(qparam); 55 } 56 57}
That’s all, after this when you call getMapper(Class modelClass)
of your KittyDatabase
implementation you would receive ready to go your extended CRUD controller.