Updating existing entity
For updating an entity with KittyORM you may use KittyMapper.save(M model)
or KittyMapper.update(M model)
method. As mentioned in the earlier lesson using save method force KittyORM to define what operation should be done with the provided model: update or insert. KittyORM makes this decision based on a state of entity fields that can be used for the unambiguous definition of associated record in the database.
When you use KittyMapper.update(M model)
method KittyORM would generate a query that sets values of a target table row with values from the model using generated UPDATE
statement with WHERE
clause. In the common case, rowid would be used for such statement. If the model has no rowid set than UPDATE
statement would be generated with WHERE
clause using model’s PK values.
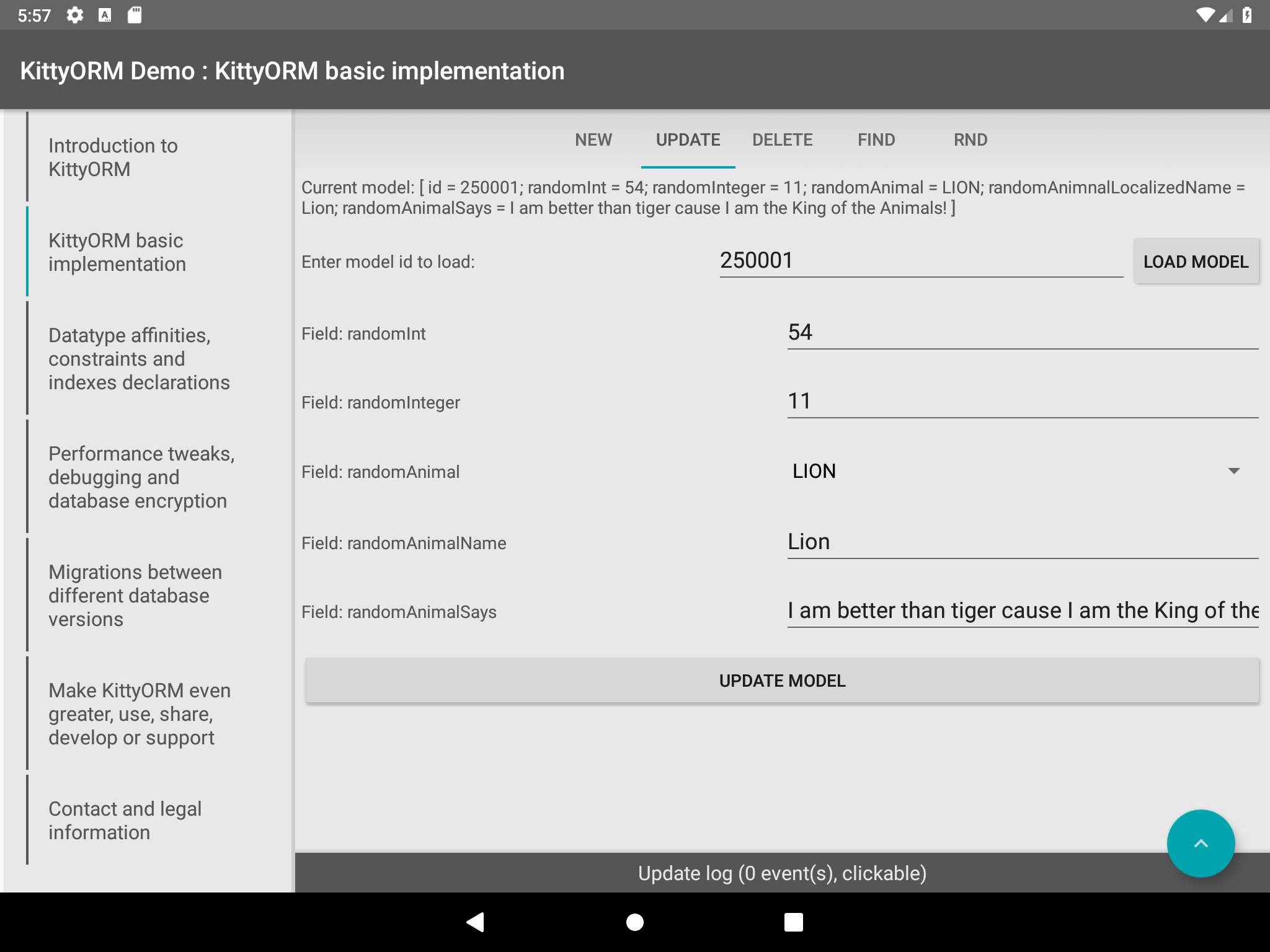
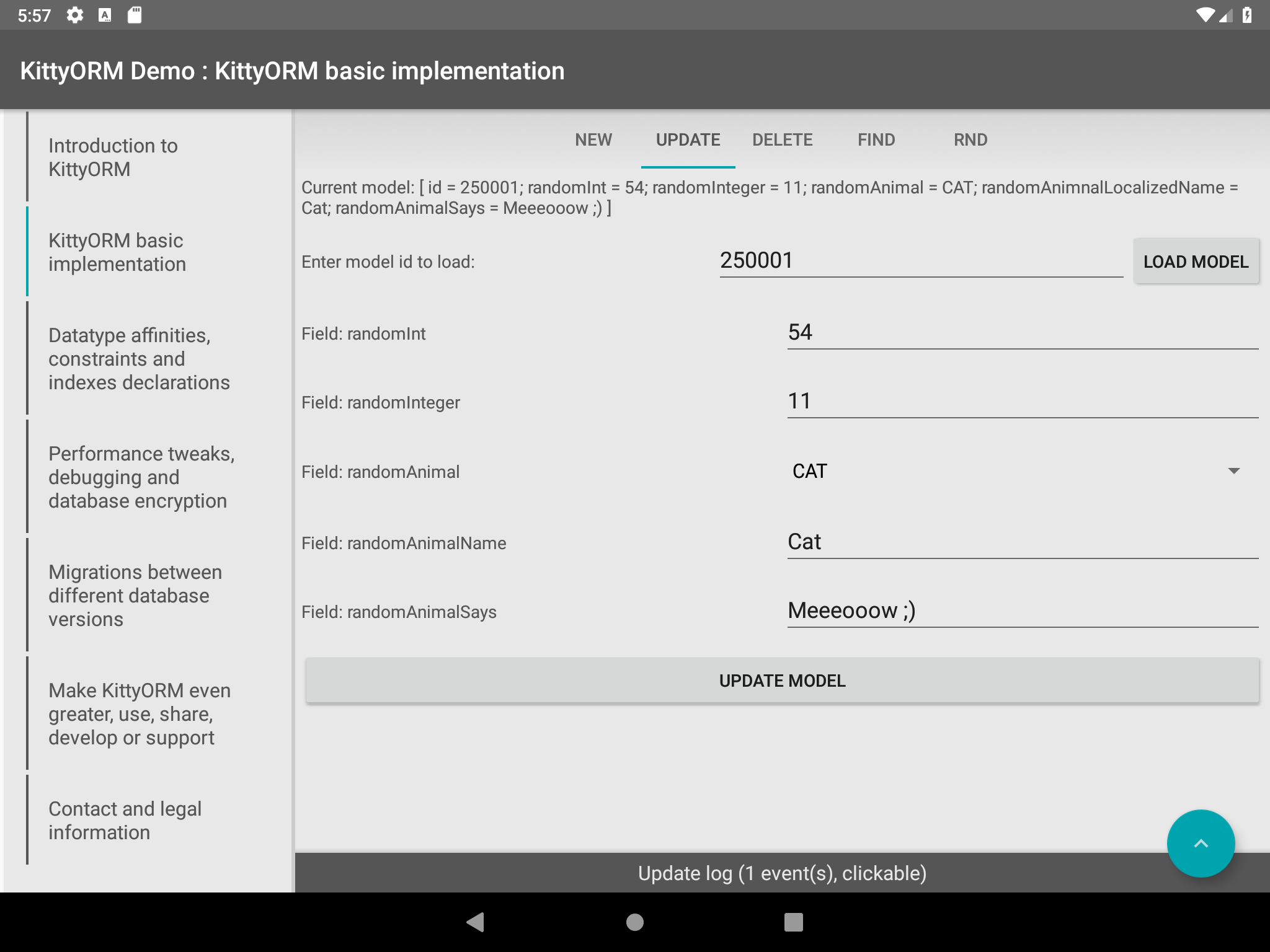
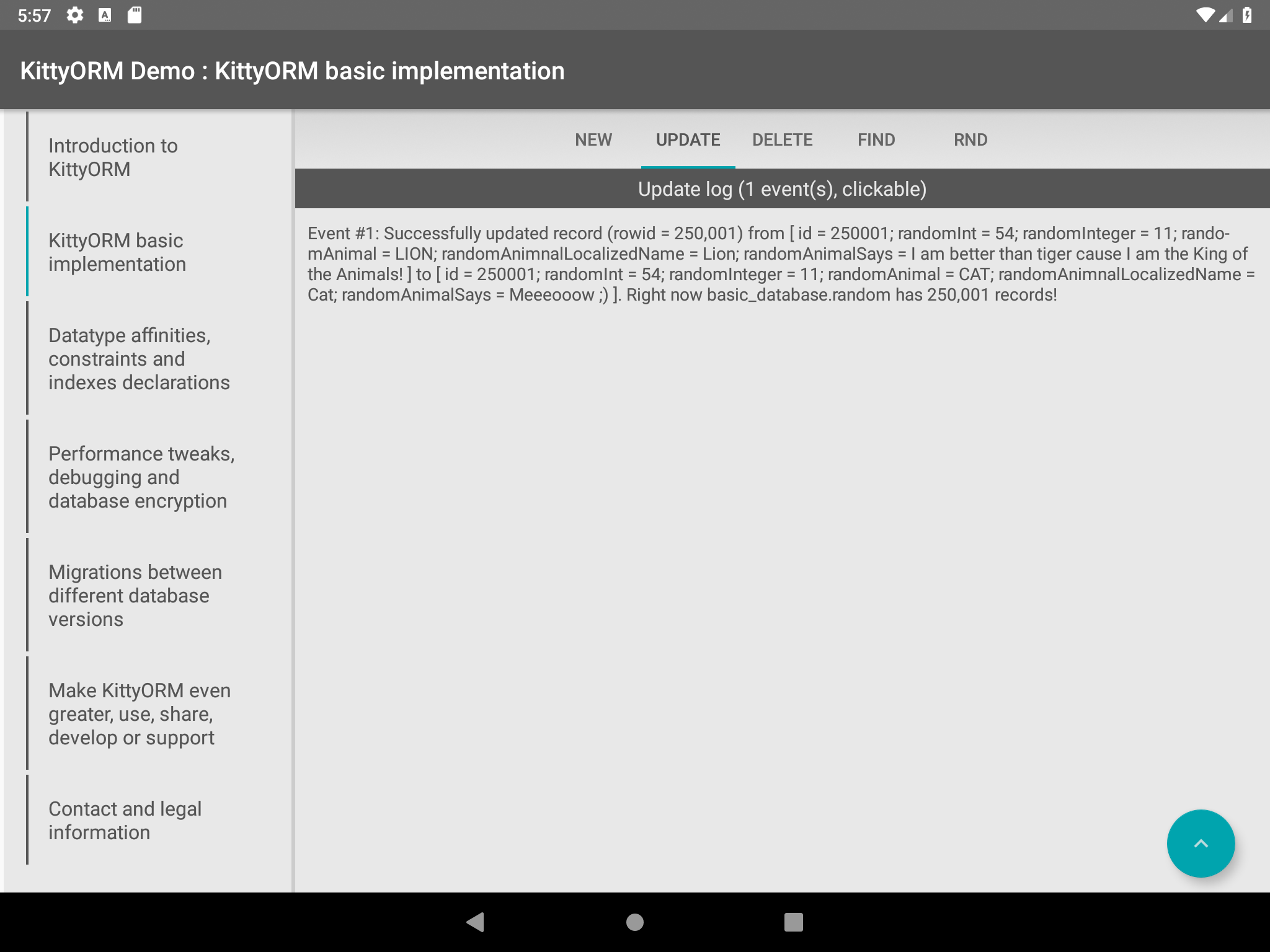
So, updating models with KittyORM is same as inserting them. Take a look at following code snippet:
1// Initializing database instance
2BasicDatabase db = new BasicDatabase(getContext());
3// Getting mapper instance
4RandomMapper mapper = (RandomMapper) db.getMapper(RandomModel.class);
5// Getting existing model from database (assuming that 0l model exists)
6RandomModel toUpdate = mapper.findByIPK(0l);
7// Setting model fields
8toUpdate.randomInt = 12;
9...
10// Saving model with save method
11mapper.save(toUpdate);
12// Saving model with direct insert call
13mapper.update(toInsert);
However, what if you want to update more than one model? It is quite simple, instead of updating models in a bulk mode you can update some rows in a table with a usage of custom update condition within values to be updated set as fields of POJO.
For example, you want to update all rows in random
table where id is between (inclusively) 10 and 20 and set a random_int column to value 50. For this perform following steps:
- Create new condition with the usage of
SQLiteConditionBuilder.class
:1SQLiteConditionBuilder builder = new SQLiteConditionBuilder(); 2builder.addColumn("id") 3 .addSQLOperator(SQLiteOperator.GREATER_OR_EQUAL) 4 .addValue(10) 5 .addSQLOperator(SQLiteOperator.AND) 6 .addColumn("id") 7 .addSQLOperator(SQLiteOperator.LESS_OR_EQUAL) 8 .addValue(20);
- Create new
RandomModel.class
instance and setrandomInt
to 50:1RandomModel toUpdate = new RandomModel(); 2toUpdate.randomInt = 50;
- Run
KittyMapper.update(M model, SQLiteCondition condition, String[] names, int IEFlag)
providing previosly created model, condition,String
array of field names (randomInt
) to set and inclusion\exclusion flag:1mapper.update(toUpdate, builder.build(), new String[]{"randomInt"}, CVUtils.INCLUDE_ONLY_SELECTED_FIELDS);
Click to view update example in a block:
1// Initializing database instance
2BasicDatabase db = new BasicDatabase(getContext());
3// Getting mapper instance
4RandomMapper mapper = (RandomMapper) db.getMapper(RandomModel.class);
5// Creating condition builder instance
6SQLiteConditionBuilder builder = new SQLiteConditionBuilder();
7builder.addColumn("id")
8 .addSQLOperator(SQLiteOperator.GREATER_OR_EQUAL)
9 .addValue(10)
10 .addSQLOperator(SQLiteOperator.AND)
11 .addColumn("id")
12 .addSQLOperator(SQLiteOperator.LESS_OR_EQUAL)
13 .addValue(20);
14// Creating blank model and setting it fields
15RandomModel toUpdate = new RandomModel();
16toUpdate.randomInt = 50;
17// Updating table with custom clause and values from model
18mapper.update(toUpdate, builder.build(), new String[]{"randomInt"}, CVUtils.INCLUDE_ONLY_SELECTED_FIELDS);
You can path to KittyMapper.update(M model, SQLiteCondition condition, String[] names, int IEFlag)
as names
parameter column fields or POJO field names. With IEFlag
you can specify how to treat values you passed as names
parameter. Following flags are supported: INCLUDE_ONLY_SELECTED_FIELDS
, INCLUDE_ALL_EXCEPT_SELECTED_FIELDS
, INCLUDE_ONLY_SELECTED_COLUMN_NAMES
, INCLUDE_ALL_EXCEPT_SELECTED_COLUMN_NAMES
and IGNORE_INCLUSIONS_AND_EXCLUSIONS
.
You can get more documentation on building clauses with SQLiteConditionBuilder.class
at KittyORM project page.